JavaScript forEach index
When looping through an array in JavaScript, it may be useful sometimes to get the index of every item in the array.
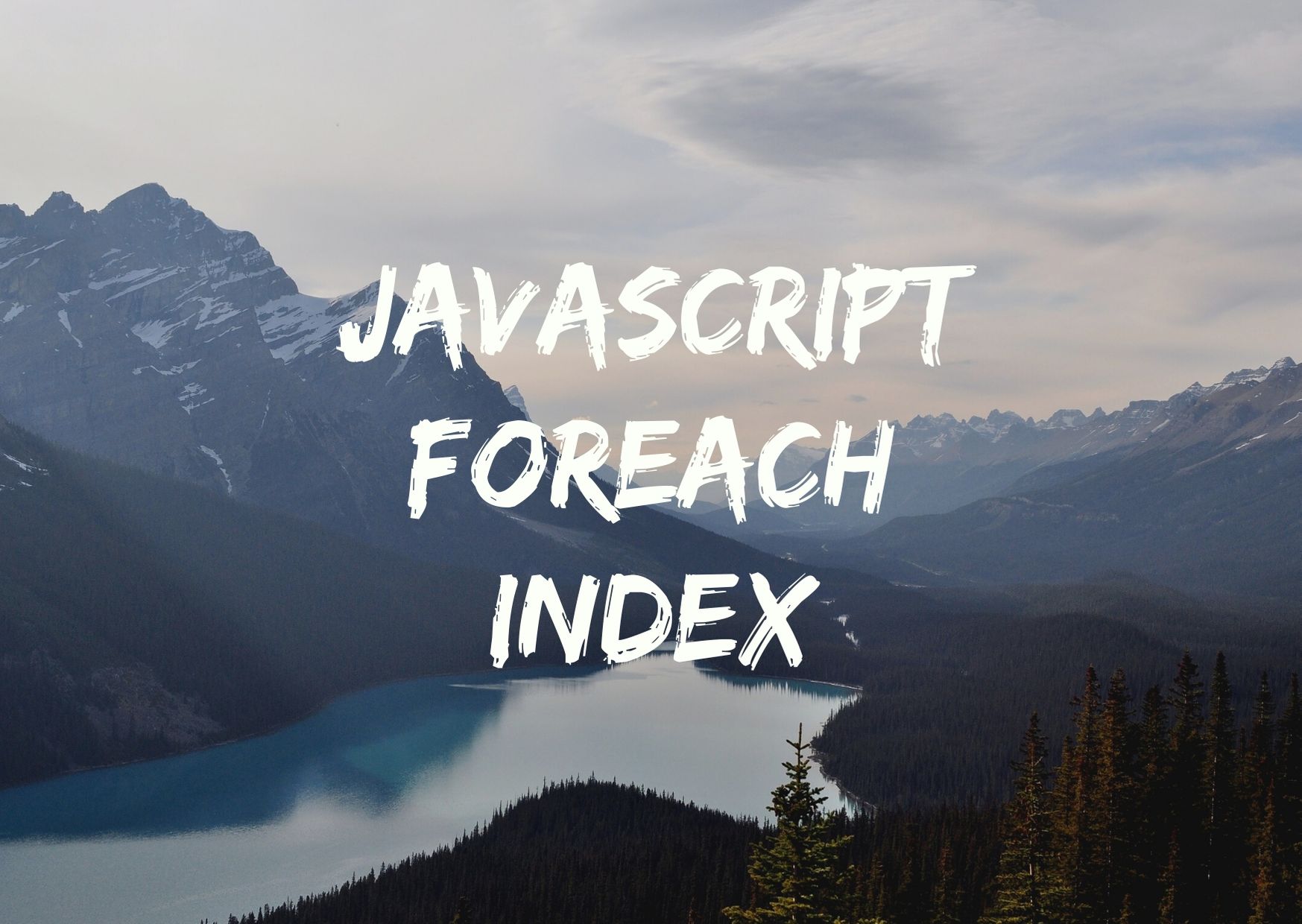
This is possible with .forEach method, let's take an example to illustrate it:
const apps = ['Calculator', 'Messaging', 'Clock'];
apps.forEach((app, index) => {
console.log(app, index);
});
The output of this code is:
"Calculator" 0
"Messaging" 1
"Clock" 2
Notice how the index starts at 0 and goes all the way until the array's length - 1 (apps.length - 1
which is 2 here).
Not sure how the forEach method works? Checkout JavaScript forEach for a full guide.
Real life scenario permalink
Why would the index
be useful? Here's a real life scenario:
Let's say you have a list of options and would like to tag them with Option 1, Option 2, etc.
const options = ['Milk', 'Cheese', 'Water'];
let html = '<div>';
options.forEach((option, index) => {
html += `<p>Select option ${index + 1}: ${option}</p>`;
});
html += `</div>`;
console.log(html);
The result of the above code is:
<div>
<p>Select option 1: Milk</p>
<p>Select option 2: Cheese</p>
<p>Select option 3: Water</p>
</div>
Notice how we added + 1
to the index
because the index is zero based (starts at zero).
Early exit permalink
Another use case of using the index
is when you want to stop the forEach once it reaches a certain index.
Note that you can't really stop the forEach but you can make it skip the body of the function. Here's an example:
const chars = ['A', 'B', 'C', 'D', 'E', 'F'];
chars.forEach((char, index) => {
if (index >= 3) {
return;
}
console.log(char);
});
The output of the above code is:
"A"
"B"
"C"
Notice how the body of the forEach is being skipped based on the index, with the condition index >= 3
Do you want to Learn JavaScript step by step?
Checkout my interactive course where you read short lessons, solve challenges in the browser and recap topics with Flashcards: